
Oh hi! This is a multi-part tutorial series, please make sure you reads the posts in order:
Part 0 - Part 1 - Part 2 - Part 3 - Part 4 - Part 5 - Part 6
So to start with, create a new file called main.go
, and write your first line:
package main
All Go programs must form a package
, which is just a way of grouping files together. If a Go program should run on its own (rather than just being referenced by another program), it needs to be in a package called main
and contain a function called main
as well (we’ll write that in a moment). If you’re coming from a procedural/scripting background, functions are just a way of organising the different bits of our program that do different things. Okay, next line!
import "net/http"
Here we’re importing the net/http
library. You may have seen similar imports in other programming langauges. The Go community has gone to the trouble of writing some excellent functions for handling HTTP requests, so we’re going to use those rather than re-invent the wheel. Importing this library lets us use all of that lovely code.
Now let’s write our first function:
func indexHandler(w http.ResponseWriter, r *http.Request) {
w.Write([]byte("<h1>Hello World!</h1>"))
}
This function is called indexHandler
. Functions in Go are defined with a name, then optionally the list of parameters or inputs they will accept in brackets, followed by - again optionally - a return type. This function is a little odd as it takes 2 inputs but returns nothing itself. The inputs are:
- an
http.ResponseWriter
assigned to the variablew
- a pointer to an
http.Request
assigned to the variabler
Please don’t worry about pointers yet!
Rather than have the function return anything, it simply accesses the http.ResponseWriter
directly. The Write
function expects some bytes, which is why we use []byte
, but don’t worry too much about that. As you can see, we’re just sending it some HTML to render the words: “Hello World!”.
Now it’s time to write our main
function. Like we said before, this is the primary function of our program and it will run when the program starts. First let’s write the skeleton of the function:
func main() {
}
Now inside the function (between the {
and }
) let’s add some more code. First:
mux := http.NewServeMux()
Here we create the variable mux
by using NewServeMux()
in the http
library. This function of the library provides us with an HTTP multiplexer, which is just a smart function that can deal with requests to different URLs and paths.
Note that :=
is just Go shorthand for creating a variable and infering it’s type at the same time. Let’s add the next line:
mux.HandleFunc("/", indexHandler)
Here we call the HandleFunc
function belonging to our mux
variable and send it 2 inputs: "/"
and indexHandler
. The HandleFunc
function sets up handlers for different URL paths. So here we’re telling it that requests for "/"
should be dealt with by the function called indexHandler
.
Now one last line for main
:
http.ListenAndServe(":8080", mux)
This calls the ListenAndServe
function that belongs to the http
library. We’re calling it directly as it’s a public function of the library, passing in a port number of our choosing to listen to, and the mux
variable we already created. This sets up an HTTP server on port 8080 and passes requests to mux
to decide where to send them. The server will continue to listen on a loop until it is terminated.
That’s it for now, so save the file. We can run this program from the terminal with:
go run main.go
Or we could build a binary with:
go build -o server main.go
And run it with:
./server
Either way, you should then be able to reach http://localhost:8080 in a browser and see your Hello World! message.
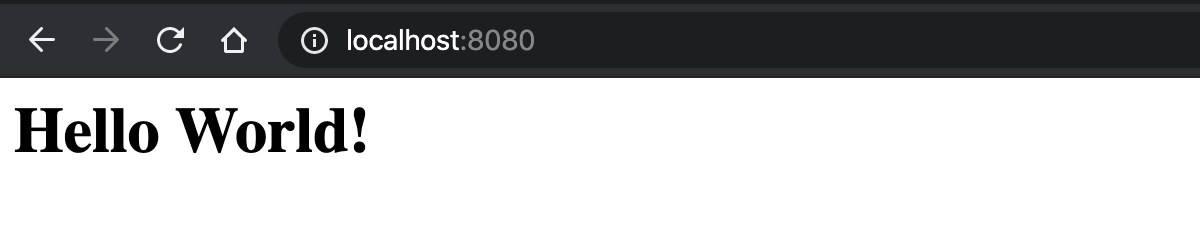
Nice work! You’ve written your first Go program :-) Now move on to part 2.
Here’s the complete code of main.go
if you need to check yours for errors: